반응형
vue 템플릿에서 많이 사용되는 내장된 디렉티브(ex. v-bind, v-for 등) 외에 사용자가 직접 Custom Directive를 생성해서 사용할 수 있다.
Simple 예시
먼저 간단한 샘플코드는 아래와 같다. directives 옵션을 사용해서 focus라는 디렉티브를 선언해주고 input 엘리먼트에서는 v-focus로 사용을 하면 된다.
<template>
<div>
<input type="text" v-focus />
</div>
</template>
<script>
const focus = {
mounted: (el) => el.focus(),
};
export default {
name: "CustomDirective",
// CustomDirective 정의
directives: {
focus,
},
};
</script>
컴포넌트를 로딩하면 아래와 같이 input에 포커스가 자동으로 적용된다.
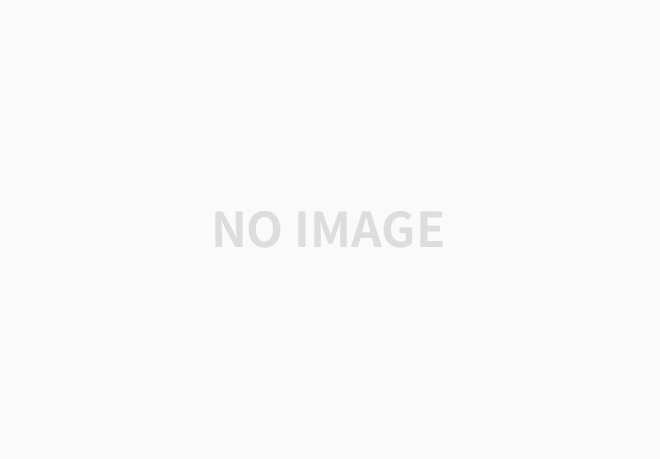
전역(Global) 설정
위에 방법은 컴포넌트 내에서 사용가능한 선언 방법이고 전역(Global) 설정을 위해서는 아래와 같이 main.js에 directive("focus", focus)로 선언을 해준다. 앞에 "v-"는 작성 할 필요없다.
const focus = {
mounted: (el) => el.focus(),
};
createApp(App)
.use(router)
.directive("focus", focus)
.mount("#app");
그리고 컴포넌트에서는 그냥 v-focus로 사용만 하면 동일하게 적용된다.
<template>
<div>
<input type="text" v-focus />
</div>
</template>
<script>
export default {
};
</script>
Directive Hook
directive 정의 객체는 아래와 같이 여러가지 훅 함수를 제공한다.
const myDirective = {
// 엘리먼트의 속성,이벤트 리스너들이 적용되기 전에 호출
created(el, binding, vnode, prevVnode) {
// see below for details on arguments
},
// 엘리먼트가 mount 되기 전 호출
beforeMount() {},
// 엘리먼트가 mount 된 후 호출
mounted() {},
// Parent컴포넌트가 update 되기 전 호출
beforeUpdate() {},
// Parent컴포넌트와 모든 자식컴포넌트가 update 된 후 호출
updated() {},
// Parent컴포넌트가 unmount 되기 전 호출
beforeUnmount() {},
// Parent컴포넌트가 unmount 된 후 호출
unmounted() {}
}
}
Directive Hook 파라메타
- el: 디렉티브가 바인딩 되는 엘리먼트, 이 것을 사용하여 DOM 조작 가능
- binding: 아래 properties를 가진 객체
- value: 디렉티브에서 전달 받은 값, ex) v-my-directive="1+1"
- oldValue: 이전 값, beforeUpdate와 updated 훅 에서만 사용 가능
- arg: 디렉티브의 argument, ex) v-my-directive:foo 에서 :뒤의 foo
- modifiers: 디렉티브 modifier ex) v-my-directive.foo 에서 .뒤의 foo
- dir: 디렉티브 정의 객체
- instance: 디렉티브가 사용된 컴포넌트 인스턴스 - vnode: vue의 Virtual Node
- prevNode: 이전의 Virtual Node, beforeUpdate와 updated 훅에서만 사용 가능
<template>
<div>
<h3 v-mycolor="'red'">Message</h3>
<!-- 정적 String을 value로 넘길경우 ''로 한번 더 감싼다 -->
<h3 v-mycolor:background="'red'">Message</h3>
</div>
</template>
<script>
const mycolor = {
mounted: (el, binding, vnode, preNode) => {
console.log(el);
console.log(binding);
console.log(vnode);
console.log(preNode);
if (binding.arg == "background") {
el.style.background = binding.value;
} else {
el.style.color = binding.value;
}
},
};
export default {
directives: {
mycolor,
},
};
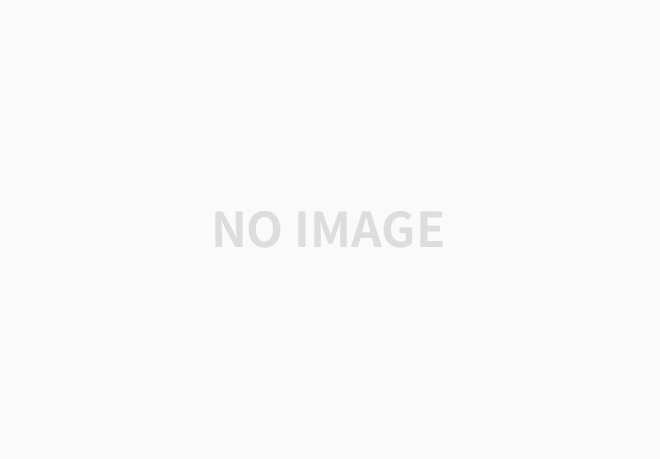
참고자료:
https://vuejs.org/guide/reusability/custom-directives.html
https://seulcode.tistory.com/265
반응형
'개발 > Vue.js' 카테고리의 다른 글
Vue.js Bootstrap - Uncaught TypeError: Cannot read properties of undefined (reading 'prototype') (0) | 2022.04.10 |
---|---|
Vue.js Vuex 알아보기 (0) | 2022.04.08 |
Vue.js Composition API 알아보기 (0) | 2022.04.03 |
Vue.js refs로 자식컴포넌트, html 엘리먼트 접근 (0) | 2022.04.02 |
Vue.js props 사용 샘플 (0) | 2022.04.02 |
댓글